Using the Mock Provider
Simulating Response Statuses
When implementing a payment flow, you will need to determine the status of an API request before proceeding to the next step in your flow. Say, for example, you are implementing a two-step Authorize-Capture flow. In this case, you should only invoke the Create Capture API if the Create Authorization API responded with a status of Succeed
. Using the mock provider, you can trigger request response statuses and verify that you handle each status correctly in your implementation.
Example: Simulating a Successful Payment Flow for Card Transactions
Let’s take a look at an example in which we simulate a successful Authorize-Capture flow. For brevity purposes, we will not show the request headers but focus on the endpoints and body data instead.
We’ll start our flow with collecting a user’s card information. The mock provider uses test card numbers to simulate a response status for authorization and charge requests. Tokenizing a card with number 5105 1051 0510 5100 will guarantee that our Create Authorization API request will be successful when called later on in the flow. Here’s an example when using the Javascript API to collect a customer’s card information:
<script>
POS.tokenize({
"token_type": "credit_card",
"holder_name": "MR C D HOLDER",
"expiration_date": "09-2019",
"card_number": "5105105105105100"
},
function (result) {
// Grab the token and pass it to your server
}
);
</script>
The next step is to create a payment by invoking the Create Payment API. The amount we’ll pass in the payment request is important, since PaymentsOS uses the amount to simulate the response status of the Create Capture API request (which we will invoke as the last step in our flow). We want PaymentsOS to simulate a successful capture, so we need to pass in an amount equal to, or higher than, USD 4000 (which is USD 40 represented in a dollar’s smallest currency unit):
var request = new XMLHttpRequest();
request.open('POST', 'https://api.paymentsos.com/payments');
...
var body = {
'amount': 4000,
'currency': 'USD',
'statement_soft_descriptor': 'Oil lamp'};
request.send(JSON.stringify(body));
curl --compressed -X POST \
https://api.paymentsos.com/payments \
...
-d '{
"amount": 1503,
"currency": "USD",
"statement_soft_descriptor": "Oil lamp"
}'
Now complete the remainder of the Authorize-Capture flow, by calling the Create Authorization API followed by the Create Capture API. Notice that the response data of the Create Capture API includes a status
key with a value of Succeed
:
{
"id": "e544612d-a46d-4ed9-aa2b-9c777f5a4dfd",
"created": "1520348674405",
"result": {
"status": "Succeed"
},
...
}
Example: Simulating a Successful Payment Flow for Transactions with Alternative Payment Methods
You can also simulate a successful for transactions done with a payment method other than cards. Needless to say, there’s no need to collect a user’s card information so just go ahead and create a payment by invoking the Create Payment API.
var request = new XMLHttpRequest();
request.open('POST', 'https://api.paymentsos.com/payments');
...
var body = {
'amount': 4000,
'currency': 'USD',
};
request.send(JSON.stringify(body));
curl --compressed -X POST \
https://api.paymentsos.com/payments \
...
-d '{
"amount": 1503,
"currency": "USD"
}'
Now invoke the Create Charge request. For Charge requests, the response status is determined by the value you pass in the payment_method.additional_details.untokenized_result_status
field. To simulate a successful response, you need to pass in value of succeed
(for other possible values, see Authorization and Charge Requests for Transactions with Alternative Payment Methods).
var request = new XMLHttpRequest();
request.open('POST', 'https://api.paymentsos.com/payments');
...
var body = {
"payment_method": {
"source_type": "ewallet",
"type": "untokenized",
"vendor": "applepay",
"additional_details": {
"untokenized_result_status": "succeed"
}
}
};
request.send(JSON.stringify(body));
curl --compressed -X POST \
https://api.paymentsos.com/payments \
...
-d '{
"payment_method": {
"source_type": "ewallet",
"type": "untokenized",
"vendor": "applepay",
"additional_details": {
"untokenized_result_status": "succeed"
}
}
}'
Simulating Response Errors
It is important that you handle any errors that may occur. PaymentsOS provides uniform error messages, regardless of the processor you transact against. We thus recommend you implement error handling for the error messages returned by PaymentsOS.
The mock provider allows you to simulate response errors by passing in specific decimal digits in the amount
field of a request body, in combination with a request that simulates a return status of Failed
.
Example: Simulating a Response Error
Let’s take a look at an example in which we simulate a provider_network_error
message when invoking an authorization request. For brevity purposes, we will not show the request headers but focus on the endpoints and body data instead.
For simulating a response error with card transactions, you need to create a token first (you can skip this step when simulating a response for transactions using an alternative payment method). So go ahead and create a token, passing in a test card number of 4242424242424242. This will cause a return status of Failed
when invoking the Create Authorization API request later on in the payment flow. Here’s an example when using the Javascript API to collect a customer’s card information:
<script>
POS.tokenize({
"token_type": "credit_card",
"holder_name": "MR C D HOLDER",
"expiration_date": "09-2019",
"card_number": "4242424242424242"
},
function (result) {
// Grab the token and pass it to your server
}
);
</script>
Now create a payment by calling the Create Payment API. A payment amount in USD of 1503 (in which the digital digits are 03) will cause a provider_network_error
when invoking the Create Authorization API request in the next step, so let’s pass it in when creating the payment:
var request = new XMLHttpRequest();
request.open('POST', 'https://api.paymentsos.com/payments');
...
var body = {
'amount': 1503,
'currency': 'USD',
'statement_soft_descriptor': 'Oil lamp'};
request.send(JSON.stringify(body));
curl --compressed -X POST \
https://api.paymentsos.com/payments \
...
-d '{
"amount": 1503,
"currency": "USD",
"statement_soft_descriptor": "Oil lamp"
}'
Finally, call the Create Authorization API (if you’re simulating a response error for transactions using an alternative payment method, make sure to pass a payment_method.additional_details.untokenized_result_status
field with a value of failed
in the request body). Then view the response data. Notice that the result
object will include a status
value of Failed
and a category
value of provider_network_error
:
{
"id": "459079bd-1d22-4068-8e21-c9fec505bc3f",
"amount": 1503,
...
"result": {
"status": "Failed",
"category": "provider_network_error",
"description": "Unable to reach the provider network."
},
...
}
Simulating Multi-Capture Behavior for Providers that do not Natively Support this Functionality
You can simulate multi-capture behavior for providers that do not natively support this functionality and for which PaymentsOS implemented such support under the hood. This allows you to invoke several capture and void requests for a single payment.
To start, you will need to set the multi-capture feature in your PaymentsOS environment to true
(Account > Providers > Mock Processor).
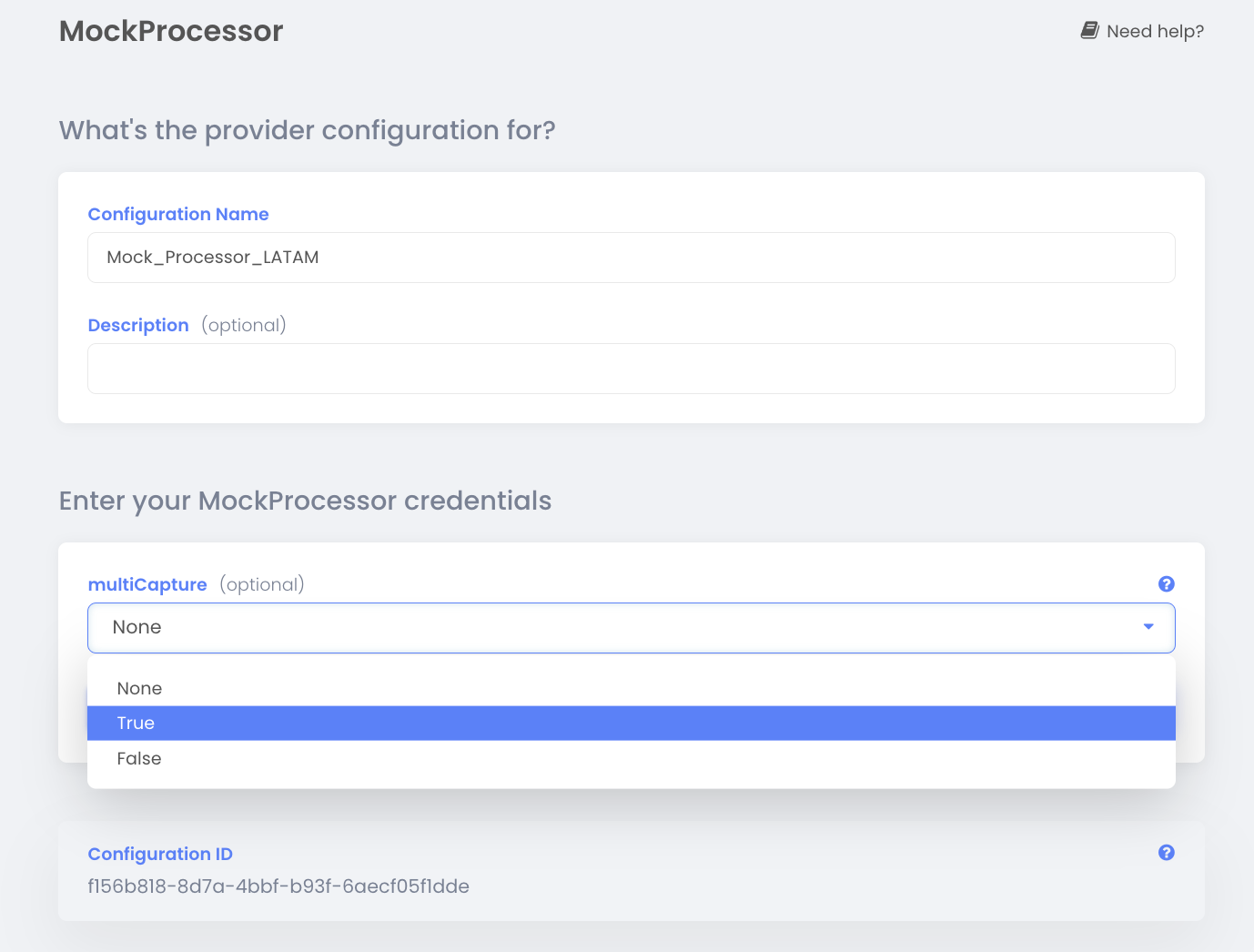
To simulate specific status outcome, pass the following test amounts in the Create Payment request according to the status you wish to simulate:
- To simulate a
succeed
status, use | 50 <= amount | - To simulate a
failed
status, use | 0 < amount < 20 |. - Note that the amount passed affects the status of all partial captures.
Next, you can invoke separate capture and void requests for every partial-capture you wish to perform.
Important Notes
- A final capture request is invoked on your behalf (under the hood) when the cumulative sum of all partial captures reaches the total payment amount, or once the time to make the final capture request has timed out (5 days).
- The status for each partial capture and void will remain
pending
until the final capture request is invoked.